Spring을 이용해 객체를 생성하는 방법은 2가지가 있다.
첫번째는 id 속성을 주고 id속성의 값을 이용해 원하는 객체를 생성하는 방법이고
두번째는 id 속성을 주지 않고 객체를 생성하는 방법이다.
아래에서 각각에 대해 알아보자
bean의 이름을 찾기(id 속성을 준 경우)
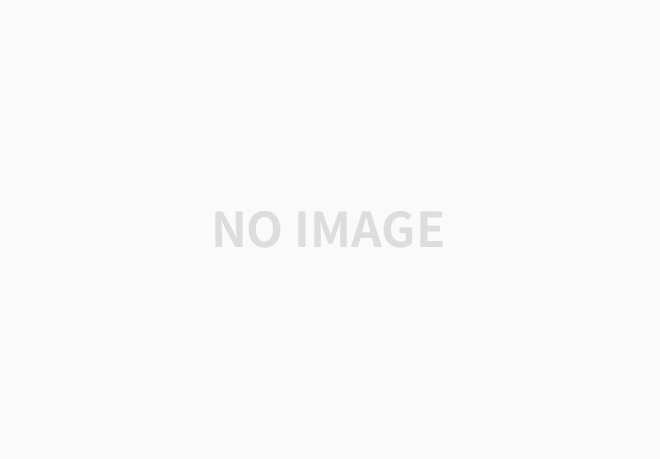
id 속성을 준 경우, id값으로 원하는 객체를 생성할 수 있다.
단, 생성된 객체는 Object type 이므로 반드시 캐스팅을 해주어야한다.
[init.xml]
Spring Bean Configuration File 을 생성 하고, 안에 핵심 객체를 생성하기 위해 정의 한다
1
2
3
4
5
6
7
8
9
|
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- 스프링이 생성해야될 목록 작성 -->
<!-- WeaponImpl 객체를 생성하고 그 객체의 이름을 myWeapon 으로 부여한다. -->
<bean id="myWeapon" class="test.mypac.TestWeapon"/>
</beans>
|
cs |
[MainClass]
MainClass에서 init.xml 문서를 해석하고 id값을 myWeapon으로 갖고 있는 객체를 생성한다.
getBean("myWeapon") 은 Object type으로 객체가 생성되기 때문에 반드시 원하는 type으로 casting을 해주어야한다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
package test.example2;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import test.mypac.Weapon;
public class MainClass {
public static void main(String[] args) {
//1. init.xml 문서를 해석해서 bean을 생성한다.
ApplicationContext context=new ClassPathXmlApplicationContext("test/example2/init.xml");
//2. 스프링이 관리하고 있는 객체중에서 "myWeapon"이라는 이름의 객체의
//참조값을 가지고 와서 weapon type으로 casting 해서 변수에 담는다.
Weapon w1=(Weapon)context.getBean("myWeapon");
//3. Weapon type의 객체를 이용해서 원하는 동작을 한다.
useWeapn(w1);
}
public static void useWeapn(Weapon w) {
w.attack();
}
}
|
cs |
bean(객체)의 type으로 찾기(id 속성을 주지 않음)
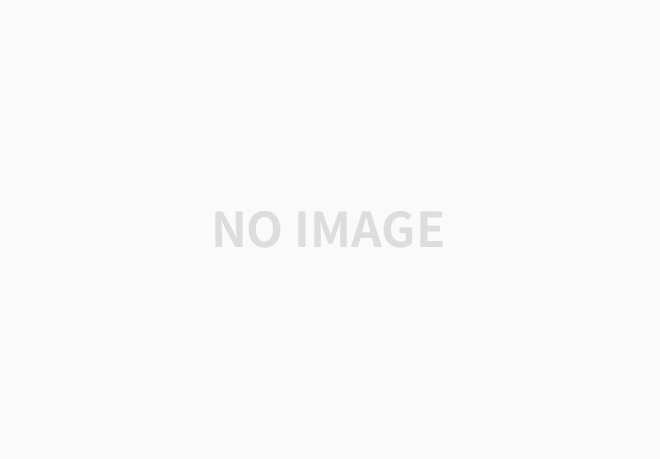
id 속성을 주지 않은 경우, bean(객체)의 type으로 생성할 객체를 찾을 수 있다.
[init.xml]
1
2
3
4
5
6
7
8
9
|
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- WeaponImpl 객체를 생성해서 관리 하도록 하고 id는 따로 부여하지 않는다. -->
<bean class="test.mypac.WeaponImpl" />
<bean class="test.example3.CarImpl" />
</beans>
|
cs |
[MainClass]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
|
package test.example3;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import test.mypac.Car;
import test.mypac.Weapon;
public class MainClass {
public static void main(String[] args) {
// test.example3 패키지에 있는 init.xml 문서를 로딩해서 해당 문서에 명시된 bean 설정에 맞게 객체를 생성하고
// 생성된 객체는 spring bean container 에서 관리한다.
ApplicationContext context=new ClassPathXmlApplicationContext("test/example3/init.xml");
//useWeapon()메소드를 호출 하려면?
//spring bean container에서 관리되는 객체중에 Weapon type의 참조값 가져오기(bean의 type으로 찾기)
Weapon w=(Weapon)context.getBean(Weapon.class);
useWeapon(w);
//useCar()메소드를 호출하려면?
Car c=(Car)context.getBean(Car.class);
useCar(c);
}
public static void useWeapon(Weapon w) {
w.attack();
}
public static void useCar(Car c) {
c.dirve();
}
}
}
|
cs |